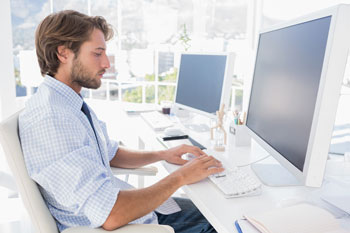
There are many things that an iOS C++ Cocos2d-x Game Developer needs to know in order to successfully develop, test and publish a game.
The following are 18 things relating to Xcode that I believe every iOS C++ Cocos2d-x Game Developer should know. Think of this article as more of an overview or cheatsheet.
To master these topics, you will likely need to do some more research. But, master each of these and your life as an iOS game developer will go much more smoothly.
Need help setting up an Cocos2d-x C++ Xcode development environment. Then checkout this article here.
Setting Up Your Account in Xcode
This is very basic but very important. After downloading Xcode from the Apple App Store, and after you have signed up for the iOS Developer Program, you should setup your developer account inside of Xcode. This can be done in the menu Xcode -> Preferences -> Accounts and then click on the plus symbol in the bottom left corner to add your Apple ID. More information can be found here.
Adding Classes and Other Code
To add a new class, from the menu you can File -> New -> File and then choose iOS -> Source -> C++ File to create a new C++ file.
To add existing classes, you can drag and drop them in folders or as individual files into the Xcode project navigator. You will then need to select which targets will use the files. That is by default the Desktop Mac target and the iOS Mobile project.
One important thing to be aware of when adding classes (and resources) to an Xcode project is that you can add by Folder Reference or create Groups. Groups are a separate structure from the file system. Folder References use the file system to create the structure seen in Xcode. But there is more to it than just how things are seen in the project navigator.
I suggest using Folder Groups when adding classes and to use Folder References for assets added to the Resources folder. I do not have a good reason for classes to use Groups, other than that is what I have seen to be more common for iOS development.
How to Structure Your Resources Folder – Groups vs. References
I do have a good reason to use Folder References for images, audio files and other assets added to the Resources folder. The reason has to do with how Cocos2d-x on Android loads assets. Cocos2d-x is a cross platform game engine and I target Android and iOS, and so should you. More about why you should target multiple platforms here.
On Android, for Cocos2d-x code to read an asset such as “Resources/SampleFolder/sound.wav”, you need to refer to it as “SampleFolder/sound.wav” in the Cocos2d-x C++ code. But adding a folder path before the file name such as SampleFolder doesn’t work in Cocos2d-x if you use Xcode Groups.
So the solution to the above problem, to get a cross platform solution, is to use Folder References in Xcode. Then I can access assets in my Cocos2d-x game code relative to the Resource folder e.g. “SampleFolder/sound.wav” and it will work as expected on Android and on iOS.
How To Rename Your iOS or Mac Project in Xcode
Renaming your project in Xcode is simple. Just click on the project name in the project navigator and then type the new name. Then confirm your change in the dialog box. You might also want to change information in the Info.plist file as well, but that is not required to change the project name.
If you change your project name, you can delete your existing scheme in the Xcode Scheme Manager and then click on “Autocreate Schemes Now” to generate a new scheme.
Schemes, Targets and Configurations
Schemes are essentially a set of targets and configurations. You can access your projects schemes in Xcode from the menu at Product -> Schemes -> Manage Schemes. The default iOS scheme from the Cocos2d-x HelloWorld template is called MY_PROJECT_NAME-mobile, where MY_PROJECT_NAME is whatever you called the project when you created it with the cocos console.
If you double-click on the MY_PROJECT_NAME-mobile scheme it will open a new dialog. This is the detail of the scheme and is called the Scheme Editor. You can also access this via the menu Product -> Scheme -> Edit Scheme.
In the upper left corner of this dialog you can select which scheme you want to view/edit. There are lots of things you can do in the Scheme editor, but the two most important probably are configuring which configuration is executed for Run and which configuration is executed for Archive.
Configurations allow you to have different build settings for different purposes. Configurations are defined at the project level and then inherited at the target level.
Cocos2d-x comes with a Debug and Release Xcode configuration. Different build settings are then applied for each configuration at the project level and then the target level can override those settings with target specific settings.
Some build settings that you should be aware of and learn more about include:
- Code Signing Identity
- Provisioning Profile
- Preprocessor Macros
- COCOS2D_DEBUG=1
- CC_ENABLE_CHIPMUNK_INTEGRATION=1
Code Signing Basics
Your code signing private key will need to be installed in your Keychain Access app on Mac to be able to sign an app. This key can be created and revoked in the Apple Developer Member Center.
You will need to use a development provisioning profile for running and testing your game on iOS devices. You will need to use an iOS App Store distribution provisioning profile for archiving and publishing your game.
Provisioning profiles are created in the Apple Developer Member Center and can be downloaded and installed by dragging them onto the Xcode icon.
Once you have your private key for signing apps and your provisioning profiles for your app, you can set which configuration uses which signing identity and profile in the build settings tab in Xcode.
Debugging Basics
For debugging in Xcode to work, start by validating your scheme settings. Open the MY_PROJECT_NAME-mobile scheme as described above in the Schemes sections. Verify that the debug configuration (because it does not strip out any symbols) is set for Run by clicking on Run on the left side of the Scheme Editor and verifying that the Build Configuration for Run is set to Debug on the right side of the Scheme Editor. Verify that the checkbox next to Debug executable is checked.
Press Command-R or alternatively press the play button to build and deploy your app for debugging.
You can set breakpoint by clicking on the left side of the class text editor, just like most other IDEs.
You can add exception breakpoints by clicking the menu View -> Navigators -> Show Breakpoint Navigator and then click the plus symbol in the bottom left corner.
Managing Game Center Config with iTMSTransporter
Creating leaderboards and achievements in iTunesConnect can be tedious if you have a lot of them. I prefer to use a python script that generates the XML file and the .itmsp package. I can then add my images for my GameCenter assets to the .itmsp package and then via command line upload the assets and definitions for Leaderboards and Achievements to iTunesConnect.
The uploading is done using Apples’ iTMSTransporter. This is definitely worth looking into if you have a lot of leaderboards or achievements and you value your time; I have cut the time down for creating leaderboards and achievements for a new game several hours to about 30 minutes using this approach.
Search Local Files and Sections of the Project
Local search is done by pressing Command->F while editing a source code file. This enables searching or replacing text in the file being edited.
Since the refactor based search and replace doesn’t work for C++ projects, the Find Navigator in the left pane in Xcode is very useful for Cocos2d-x project search and replace when changes need to occur in more than just one file.
Care must be taken when using search-and-replace, because the scope is not checked on your replacements. You can narrow the scope yourself by clicking just below the search text field and then highlight the desired folders to search in the Find Navigator. You can then click on the Find text above the search text field to change from Find to Replace.
In addition to Text search, you can also do a Reference or Definitions search. This can also help narrow the scope of your search/replace.
You can even use regular expressions for your search criteria.
General Settings Tab
This is where you change the Bundle Identifier, version number, build number, deployment target, and can add/remove frameworks. To view the General Settings, click on the project name in the project navigator, then on the target MY_PROJECT_NAME-mobile, and then on the General tab on the top.
Use Relative Paths
If you share your project with others or work from multiple computers, then using relative paths is a must.
When defining header search paths, Library Search Paths, or Framework Search Paths in the build settings, it is a good idea to use relative paths. You can use the variable $(SRCROOT) to define a relative path from the source root. The source root is where the MY_PROJECT_NAME.xcodeproj file is located.
Header, Library and Framework Search Paths
You might need to add header, library or framework search paths if you use third party libraries such as Admob or others. This is easy to do. Just open the Build Settings of the target (Project Navigator, click on project name, click on target name, click on build settings) and then in the search box in the upper right corner type in search paths. You will then see the location in the build settings for the Header Search Paths, Library Search Paths, and Framework Search Paths. These can then be defined for each configuration.
Linker Flags
Some third-party libraries will require that you use -all_load as a linker flag. But these can cause problems for other libraries in you Cocos2d-x game. To meet the requirement of the third-party library and not break other functionality in your app, you should always prefer to use -force_load for the specific third-party library, rather than -all_load for the entire codebase. This is done like this:
-force_load $(SRCROOT)/../PATH_TO_LIB.a
Downloading and Installing Simulators, Command Line Tools, etc.
In the menu Xcode -> Preferences -> Downloads you can select which documentation and simulators are installed on your computer.
Icons and Splash Screens
It can be challenging to get Xcode Asset Catalogs to work properly. I have’t read extensive documentation on how to use them, but it definitely is not intuitive. After about an hour of searching and reading I decided to go with a way that I know works. I ensure the following icon and slash screens are in the pro.ios_mac/ios folder:
Icon-29.png
Icon-40.png
Icon-50.png
Icon-57.png
Icon-58.png
Icon-72.png
Icon-76.png
Icon-80.png
Icon-87.png
Icon-100.png
Icon-114.png
Icon-120.png
Icon-144.png
Icon-152.png
Icon-180.png
Default-568h@2x.png
Default-667h@2x.png
Default-736h@3x.png
Default.png
Default@2x.png
Default-Landscape~ipad.png
Note that all default splash screen images are portrait, except the one for iPad.
I then remove all references to UILaunchImages in the Info.plist file.
Then I add the following to the Info.plist and be sure to remove any existing icon references.
<key>CFBundleIconFiles</key> <array> <string>Icon-29</string> <string>Icon-40</string> <string>Icon-50</string> <string>Icon-57</string> <string>Icon-58</string> <string>Icon-72</string> <string>Icon-76</string> <string>Icon-80</string> <string>Icon-87</string> <string>Icon-100</string> <string>Icon-114</string> <string>Icon-120</string> <string>Icon-144</string> <string>Icon-152</string> <string>Icon-180</string> </array> <key>CFBundleIconFiles~ipad</key> <array> <string>Icon-29</string> <string>Icon-40</string> <string>Icon-50</string> <string>Icon-57</string> <string>Icon-58</string> <string>Icon-72</string> <string>Icon-76</string> <string>Icon-80</string> <string>Icon-87</string> <string>Icon-100</string> <string>Icon-114</string> <string>Icon-120</string> <string>Icon-144</string> <string>Icon-152</string> <string>Icon-180</string> </array>
You can change the name that is under the icon of your game. To do this, click on the project name in the project navigator, then on the target MY_PROJECT_NAME-mobile, and then on the Info tab. On the left is a key called Bundle display name. You can change the value from its default which is the variable ${PRODUCT_NAME} to whatever you want.
Build Number and Build Version Short String
Each time you submit an update of you app, you will need to increment the Build number defined in the General tab. The build number that is called Build in the General tab is the same as the value you can set in the Info.plist for the key “Bundle version”. This number can be something like 1.0, or 1.0.0.
You also have a display string value that does not need to but probably should be the same or similar to your Build number. This is called Version in the General tab and is the same value you can enter for “Bundle versions string, short” in the Info.plist file.
Archiving for Distribution
Before archiving, open the Scheme for the game you want to archive as described above in the Scheme section. Click Archive on the left and then verify which configuration is set for archiving. By default it is the Release configuration.
I prefer to duplicate the release configuration and call the new configuration Distribution. Then I assign that configuration for Archiving. To duplicate a configuration, click on the project name in the project navigator, then click on the project name in the pane to the right and then click on the Info tab. You can click on the Release configuration and then click the plus sign below the configuration and select duplicate.
After you have selected the configuration you want to use for archiving, go to the build setting for that configuration by clicking on the project name in the project navigator, then the target name and then build settings. Verify that you have the code signing configured as desired. Also, review the other build settings to see if there is anything that might not be set as expected.
If you make changes to the Distribution configuration, it is important to immediately make those changes to the Release configuration. This way your build and release configurations stay in sync. If I made changes frequently to the build settings, it probably would be better to just have a Debug and a Release configuration and then change the code signing when I want to archive. But since I rarely make changes to the build settings, I am comfortable having separate configurations of Release and Distribution.
To Archive your game, from the menu select Product -> Archive. If Archive is greyed out, then you need to ensure that you have an archivable scheme selected. To the right of the play and stop buttons you can click on a dropdown list and make sure that you have MY_PROJECT_NAME-mobile selected. Then you need to ensure you have iOS device selected to the right of that. You cannot archive for some reason if the target device is set to a simulator.